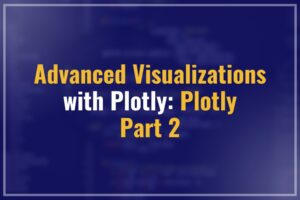
Welcome to the next part of our NumPy series! In this exciting Part 3, we’re delving deeper into the world of NumPy arrays, uncovering advanced manipulation techniques and powerful operations that will elevate your data handling and analysis skills. So, let’s transition seamlessly into the heart of NumPy’s capabilities, where you’ll learn to slice, reshape, concatenate, and perform intricate operations with ease. Get ready to unleash the full potential of NumPy as we explore these advanced topics step by step.
Â
In this section, we delve into the fundamental concepts of indexing and slicing in NumPy arrays. These techniques provide you with precise control over your data, allowing you to access and manipulate it with ease. Whether you’re a novice or an experienced data wrangler, mastering these skills is essential. So, let’s embark on this journey, transitioning from the basics to advanced slicing strategies, and discover how to navigate your arrays effectively, extracting the information you need seamlessly.
In this section, we will focus exclusively on indexing techniques in NumPy, from the most basic to more advanced methods. Proper understanding and mastery of these techniques are fundamental for efficient data retrieval and manipulation.
Q: What is Indexing in NumPy, and How Does It Work?
A: Indexing in NumPy is the process of selecting specific elements or subsets of data from a NumPy array. It allows you to precisely locate and retrieve information based on the position or conditions defined by indices or boolean masks. Indexing is a fundamental technique that facilitates data manipulation, enabling tasks like accessing individual elements, extracting rows and columns in 2D arrays, and filtering data based on specific criteria. By leveraging indexing, you gain fine-grained control over your data, making it a fundamental skill for efficient data handling in NumPy arrays.
Basic indexing represents the foundational approach for interacting with NumPy arrays actively. It empowers you to retrieve specific elements within an array, forming the cornerstone for advanced data manipulation techniques.
Â
Within NumPy, arrays stand as meticulously ordered data collections, where each element occupies a distinct position or index. It’s paramount to recognize Python’s zero-based indexing convention: the first element resides at index 0, the second at index 1, and so forth.
In this illustrative case, our array arr
contains elements [10, 20, 30, 40, 50]
. To access specific elements, we employ square brackets []
, followed by the index of the desired element. In this instance, arr[1]
enables us to obtain the second element, yielding 20
.
Â
Understanding this basic indexing procedure serves as the essential springboard for conducting more intricate operations such as slicing, filtering, and reshaping arrays. Proficiency in accessing individual elements is pivotal for adeptly navigating and manipulating data housed within NumPy arrays.
In this illustrative case, our array arr
contains elements [10, 20, 30, 40, 50]
. To access specific elements, we employ square brackets []
, followed by the index of the desired element. In this instance, arr[1]
enables us to obtain the second element, yielding 20
.
Â
Understanding this basic indexing procedure serves as the essential springboard for conducting more intricate operations such as slicing, filtering, and reshaping arrays. Proficiency in accessing individual elements is pivotal for adeptly navigating and manipulating data housed within NumPy arrays.
Slicing is an essential skill when working with 2D arrays in NumPy, enabling us to extract specific rows and columns efficiently. This technique extends our data manipulation capabilities and is pivotal for data analysis and processing tasks.
In a 2D array, we have rows and columns, forming a grid-like structure. Each element resides at the intersection of a specific row and column, and we can access them by specifying their respective indices. To perform row or column slicing, we employ basic indexing techniques.
In this instructive example, we delve into 2D array slicing. We first extract the second row from the matrix
using matrix[1, :]
. This gives us [4, 5, 6]
, representing the entire second row. Next, we slice the first two columns with matrix[:, :2].
Advanced indexing represents a powerful technique within NumPy, enabling us to filter data dynamically based on specific conditions using boolean masks. This approach is invaluable for selective data extraction and manipulation in complex datasets.
Â
Boolean indexing hinges on the creation of boolean masks – arrays of True
and False
values – that indicate which elements in an array satisfy particular criteria. By applying these masks, we can extract only the data that meets our defined conditions.
Â
In this illustrative instance, we employ boolean indexing to filter data. We first create a boolean mask mask
that identifies values greater than 30 within the data
array. Subsequently, we apply this mask to data
, resulting in filtered_data
, which includes values [40, 50]
.
Â
Boolean indexing empowers us to extract and manipulate data with precision, making it a fundamental tool for data analysis and selection tasks. It allows us to work with data dynamically, adapting to specific conditions or criteria, and is a key skill in advanced data exploration.
Within advanced indexing, integer array indexing offers a versatile means of accessing elements based on specific index values. This technique allows us to precisely pinpoint and retrieve data points within an array, enhancing our control over data manipulation.
Integer array indexing revolves around providing an array of integers as indices, instructing NumPy to select elements corresponding to these integer positions.
In this exemplar, integer array indexing comes into play. We establish an array of indices named indices
containing [1, 3]
. By applying these indices to the data
array, we extract the elements at positions 1 and 3, yielding selected_elements
as [20, 40]
.
Integer array indexing serves as a robust tool for targeted data extraction and manipulation. It empowers us to access specific data points efficiently, making it a valuable technique for various analytical and processing tasks.
Array slicing in NumPy is a versatile and essential technique for both subsetting and modifying data within arrays. This powerful tool allows you to precisely select portions of an array, making it indispensable for various data manipulation tasks.
Slicing permits the extraction of specific segments from an array based on defined indices or ranges. It empowers you to focus on particular rows, columns, or individual elements, enabling fine-grained data analysis.
Example:
Consider a scenario where we have a 2D NumPy array matrix
, and we want to extract a specific portion of it. In this example, we will subset rows and columns.
In the above code, we utilize array slicing to extract a subset of the matrix
. The slicing matrix[1:3, 0:2]
signifies that we want rows 1 and 2 (inclusive) and columns 0 and 1 (inclusive). As a result, subset
will contain the values [[4 5] [7 8]]
.
Slicing is not limited to extraction; it also facilitates effortless data modification within an array. You can assign new values to selected elements or segments, updating the array in place to reflect your changes.
Example:
Now, let’s explore how to modify data within a NumPy array using array slicing.
In this code, we have a 1D NumPy array data
. Using array slicing data[1:4]
, we target elements from index 1 to 3 (inclusive). We then assign new values [22, 33, 44]
to this slice, resulting in data
being modified to [10, 22, 33, 44, 50]
.
Array slicing thus serves a dual role, allowing for precise data extraction and efficient in-place data modification. Mastery of this technique is pivotal for effective data manipulation in NumPy arrays, making it a foundational skill for data analysis and manipulation tasks.
Array concatenation and splitting are fundamental operations in NumPy, empowering you to manipulate and manage data effectively. In this section, we’ll delve into methods for combining arrays both vertically and horizontally through concatenation. Additionally, we’ll explore techniques to split arrays into smaller, more manageable pieces.
Array concatenation is a crucial operation in NumPy, allowing you to combine multiple arrays to create a larger, more comprehensive one. NumPy offers versatile functions for concatenation, providing flexibility in how you merge arrays.
Vertical concatenation, often referred to as stacking, actively involves stacking arrays on top of each other along the vertical axis. This operation effectively increases the number of rows in the resulting array, allowing you to combine data from different sources seamlessly. Here’s a detailed explanation along with an example.
Â
For instance, if you have two arrays, array1
and array2
, you can vertically concatenate them as follows:
Let’s demonstrate vertical concatenation using two arrays, array1
and array2
, each containing data for two distinct groups of people, including their ages and heights:
To consolidate data for all individuals into a single array, we actively apply vertical concatenation.
The vertical_concatenated
array now actively contains the combined data for all individuals from both groups:
This active operation demonstrates how vertical concatenation actively expands datasets along the vertical axis, enabling comprehensive data integration.
Horizontal concatenation, in contrast, actively entails merging arrays side by side along the horizontal axis. This operation actively augments the number of columns in the resulting array, making it a valuable technique for combining data from different sources when you need to extend the dataset along the horizontal dimension.
In NumPy, you can actively perform horizontal concatenation using the np.hstack()
function. It actively takes a tuple of arrays to concatenate horizontally and actively returns the resulting array.
Let’s actively illustrate horizontal concatenation with a practical example. Consider two arrays, array1
and array2
, representing data for two different groups of people, each containing their ages and heights:
This actively demonstrates how horizontal concatenation enhances datasets by adding columns along the horizontal axis, facilitating comprehensive data integration.
Array splitting actively represents the reverse operation in NumPy, where you actively break a larger array into smaller, more manageable segments. This active procedure plays a pivotal role when you actively need to disassemble data into specific, digestible portions for detailed analysis, focused processing, or targeted operations. NumPy actively equips you with functions that actively facilitate this task with precision and adaptability.
Vertical splitting, also recognized as splitting along the vertical axis, actively revolves around dividing a larger array into smaller arrays vertically. This active process effectively generates sub-arrays with fewer rows, a valuable technique when you actively require data separation based on specific criteria or intend to partition datasets for parallel processing.
Syntax:
In NumPy, vertical splitting actively leverages the np.vsplit()
function. This active function actively necessitates the following inputs:
Conversely, horizontal splitting actively revolves around segmenting a larger array into smaller arrays horizontally. This active operation actively generates sub-arrays with fewer columns, a valuable approach when you actively seek to isolate specific attributes or features within a dataset or wish to compartmentalize data for individualized analysis.
Syntax:
For horizontal splitting, NumPy actively provides the np.hsplit()
function. This active function actively requires the following inputs:
These active array splitting techniques serve as essential tools when you actively need to disassemble data for focused analysis, streamline parallel processing, or undertake other specific tasks. They actively empower you to dissect and manage large datasets with precision and ease.
Active array reshaping is a fundamental operation in NumPy that enables you to alter the structure of an array while preserving its elements. This active capability is crucial when adapting data for operations like matrix multiplication or when changing array dimensions for specific tasks.
In NumPy, active reshaping is facilitated by the np.reshape()
method or the .reshape()
method of an array. You actively specify the new shape, and NumPy adjusts the elements accordingly.
Let’s actively illustrate array reshaping with an example. Suppose you have a one-dimensional array data
containing temperature readings for different days, and you want to reshape it into a two-dimensional array with rows representing weeks and columns representing days.
Now, reshaped_data
actively holds the data. This active reshaping allows you to effectively organize temperature data for further analysis.
Â
Active transposition involves switching the rows and columns of a 2D array. This active operation is particularly significant in matrix operations, where you actively manipulate arrays for tasks like matrix multiplication or finding the transpose of a matrix.
Â
In NumPy, active transposition is achieved using the .T
attribute of an array or the np.transpose()
function.
Understanding these active reshaping and transposition capabilities is essential for effectively manipulating and analyzing data in various scenarios.
Active transposition is crucial in matrix operations. Suppose you have a 2D array matrix
representing a rotation matrix, and you want to find its transpose to invert the rotation.
Now, transposed_matrix
actively contains the data. This active transposition allows you to effectively manipulate matrices for various mathematical operations.
In conclusion, this blog has provided a comprehensive exploration of NumPy arrays, covering essential topics such as array creation, indexing, slicing, advanced indexing, and array reshaping. We have actively learned how to create, manipulate, and extract data from arrays, gaining a solid understanding of NumPy’s powerful capabilities.
In the upcoming sections of this series, we will actively continue to explore more advanced topics in NumPy, allowing you to further enhance your proficiency in numerical computing and data analysis using Python. Stay tuned and follow 1stepgrow for more active insights into the world of NumPy!
We provide online certification in Data Science and AI, Digital Marketing, Data Analytics with a job guarantee program. For more information, contact us today!
Courses
1stepGrow
Anaconda | Jupyter Notebook | Git & GitHub (Version Control Systems) | Python Programming Language | R Programming Langauage | Linear Algebra & Statistics | ANOVA | Hypothesis Testing | Machine Learning | Data Cleaning | Data Wrangling | Feature Engineering | Exploratory Data Analytics (EDA) | Â ML Algorithms | Linear Regression | Logistic Regression | Decision Tree | Random Forest | Bagging & Boosting | PCA | SVM | Â Time Series Analysis | Natural Language Processing (NLP) | NLTK | Deep Learning | Neural Networks | Computer Vision | Reinforcement Learning | ANN | CNN | RNN | LSTM | Facebook Prophet | SQL | MongoDB | Advance Excel for Data Science | BI Tools | Tableau | Power BI | Big Data | Hadoop | Apache Spark | Azure Datalake | Cloud Deployment | AWS | GCP | AGILE & SCRUM | Data Science Capstone Projects | ML Capstone Projects | AI Capstone Projects | Domain Training | Business Analytics
WordPress | Elementor | On-Page SEO | Off-Page SEO | Technical SEO | Content SEO | SEM | PPC | Social Media Marketing | Email Marketing | Inbound Marketing | Web Analytics | Facebook Marketing | Mobile App Marketing | Content Marketing | YouTube Marketing | Google My Business (GMB) | CRM | Affiliate Marketing | Influencer Marketing | WordPress Website Development | AI in Digital Marketing | Portfolio Creation for Digital Marketing profile | Digital Marketing Capstone Projects
Jupyter Notebook | Git & GitHub | Python | Linear Algebra & Statistics | ANOVA | Hypothesis Testing | Machine Learning | Data Cleaning | Data Wrangling | Feature Engineering | Exploratory Data Analytics (EDA) | Â ML Algorithms | Linear Regression | Logistic Regression | Decision Tree | Random Forest | Bagging & Boosting | PCA | SVM | Â Time Series Analysis | Natural Language Processing (NLP) | NLTK | SQL | MongoDB | Advance Excel for Data Science | Alteryx | BI Tools | Tableau | Power BI | Big Data | Hadoop | Apache Spark | Azure Datalake | Cloud Deployment | AWS | GCP | AGILE & SCRUM | Data Analytics Capstone Projects
Anjanapura | Arekere | Basavanagudi | Basaveshwara Nagar | Begur | Bellandur | Bommanahalli | Bommasandra | BTM Layout | CV Raman Nagar | Electronic City | Girinagar | Gottigere | Hebbal | Hoodi | HSR Layout | Hulimavu | Indira Nagar | Jalahalli | Jayanagar | J. P. Nagar |Â Kamakshipalya | Kalyan Nagar | Kammanahalli | Kengeri | Koramangala | Kothnur | Krishnarajapuram | Kumaraswamy Layout | Lingarajapuram | Mahadevapura | Mahalakshmi Layout | Malleshwaram | Marathahalli | Mathikere | Nagarbhavi | Nandini Layout | Nayandahalli | Padmanabhanagar | Peenya | Pete Area | Rajaji Nagar | Rajarajeshwari Nagar | Ramamurthy Nagar | R. T. Nagar | Sadashivanagar | Seshadripuram | Shivajinagar | Ulsoor | Uttarahalli | Varthur | Vasanth Nagar | Vidyaranyapura | Vijayanagar | White Field | Yelahanka | Yeshwanthpur
Mumbai | Pune | Nagpur | Delhi | Gurugram | Chennai | Hyderabad | Coimbatore | Bhubaneswar | Kolkata | Indore | Jaipur and More