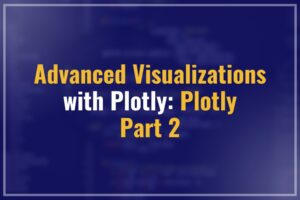
Welcome to our Python series, where we dive deep into the world of Python programming! If you’re just joining us, be sure to check out our previous posts on topics like operators and data types. In this installment, we’re going to take a closer look at one of the most important concepts in programming: conditional statements.
Conditional statements in Python enable you to create logical pathways in your code that can make decisions based on specific conditions. For example, you might want to run a certain block of code only if a particular variable is equal to a certain value. In Python, there are several different types of conditional statements, each with its own syntax and use cases.
Â
In this blog, we’ll walk you through each type of conditional statement in Python, from the basic “if” statement to the more complex “if-elif-else” statement. We’ll give you plenty of examples to help you understand how each statement works, and we’ll also cover some tips for when to use each type of statement in different programming situations.
Â
So, whether you’re a beginner just starting to learn Python or an experienced programmer looking to refresh your knowledge, you’re sure to find something valuable in this guide to Python conditional statements. Let’s dive in!
In Python, there are several different types of conditional statements that you can use to make decisions in your code based on certain conditions.Â
The most basic type is the “if” statement, which allows you to run a certain block of code only if a particular condition is true.
The “if-else” statement is similar to the “if” statement, but it also allows you to run a different block of code if the condition is false.
Â
The “if-elif-else” statement is a more complex type of conditional statement that allows you to check multiple conditions and run different blocks of code based on each condition.
Â
Finally, the ternary operator is a shorthand way of writing a simple “if-else” statement in a single line of code.
Â
In this blog, we’ll dive deeper into each type of conditional statement and show you how to use them effectively in your Python code.
Â
Â
Conditional statements are a powerful tool in Python programming, allowing us to make decisions based on certain conditions. One of the most basic types of conditional statements in Python is the “if” statement. With an “if” statement, we can run a certain block of code only if a specific condition is met. Let’s take a closer look at how to use “if” statements in Python.
Â
The syntax for an ‘if’ statement in Python is relatively straightforward and easy to understand. We start with the “if” keyword, followed by the condition we want to check, and end the line with a colon. Then, we indent the code we want to run if the condition is true.Â
Â
Syntax:
if condition:
# code to run if the condition is True
Â
Let’s break down the different parts of the ‘if’ statement syntax, so you can better understand how it works.Â
Â
Â
Â
Â
Â
It’s important to note that the code that runs if the condition is True must be indented to the right of the “if” statement. This tells Python that the indented code is inside the “if” block, and should only be executed if the condition is True.
Example Code:
In this example, we’re checking whether the temperature is greater than 90 degrees. If it is, we print the message “It’s a hot day!” to the console. If the temperature is not greater than 90 degrees, the code inside the “if” statement is skipped.
Â
One thing to note is that the condition we’re checking must evaluate to a boolean value (either True or False). In the example above, the condition “temperature > 90” is a boolean expression, so it works well with the “if” statement.
Â
Overall, “if” statements are a great way to add decision-making capabilities to your Python code. They allow you to control the flow of your program and make it more flexible and responsive to different situations.
Â
Â
Sometimes, we need to check multiple conditions in an “if” statement. For example, we might want to check if a number is both greater than 5 and less than 10. This is where logical operators like “and” and “or” come in handy. They allow us to combine multiple conditions in a single “if” statement. Let’s take a closer look at how to use these logical operators in Python.
Â
The ‘and’ operator in Python will only return True if both conditions being evaluated are True. The “or” operator, on the other hand, returns True if at least one condition is True.Â
Here’s an example:
In this example, we’re checking whether the temperature is greater than 90 degrees and the humidity is less than 50%. If both conditions are True, we print the message “It’s hot and dry outside!” to the console.
Â
We can also use the “or” operator to check if at least one of two conditions is True. Here’s an example:
In this example, we’re checking whether the temperature is greater than 90 degrees or the humidity is less than 50%. If either condition is True, we print the message “It’s either hot or dry outside!” to the console.
Â
Overall, using logical operators in “if” statements can make our code more flexible and allow us to check multiple conditions at once. They’re a great tool to have in your Python programming toolbox!
Â
Â
What if we want our program to do one thing if a condition is true, and another thing if the condition is false? This is where “if-else” statements come in handy. They allow us to create different pathways in our code, depending on whether a condition is true or false. Let’s take a closer look at how to use “if-else” statements in Python.
Â
The basic structure of an “if-else” statement looks like this:
if condition:
# Code to execute if condition is True
else:
# Code to execute if condition is False
Â
Here’s an example that uses an “if-else” statement to check if a number is even or odd:
In this example, we use the modulo operator (%) to check if the number is even or odd. If the number is even, the code inside the “if” block is executed and we print the message “The number is even!”. If the number is odd, the code inside the “else” block is executed instead and we print the message “The number is odd!”.
Â
Overall, “if-else” statements are a powerful tool that can help us create more complex logic in our programs. With them, we can make our code more flexible and responsive to different conditions!”
In this example, we use an “if-else” statement with multiple “elif” (short for “else if”) statements to assign a letter grade based on a student’s numerical grade. If the student’s grade is above 90, we print “A”. If the grade is between 80-89, we print “B”. If the grade is between 70-79, we print “C”. Otherwise, if the grade is below 70, we print “Fail”.
Â
Â
Have you ever had to make a decision based on multiple conditions, where each condition has its own set of outcomes? That’s where nested “if-else” statements come in handy!
Â
Nested “if-else” statements allow us to create more complex conditional logic in our programs by building upon the basic structure of “if-else” statements. In a nested “if-else” statement, we include another “if-else” statement inside the code block of either the “if” or “else” block of the outer “if-else” statement.
Â
Let’s take a look at an example. Imagine you’re grading a student’s exam, and you need to decide whether they passed or failed the class. But wait, there’s more! You also want to give the student the option to retake the exam if they failed, but you need to make sure they actually want to retake it. That’s where a nested “if-else” statement can help:
In this example, we start with the outermost condition: if the student’s grade is above or equal to 60, they pass the class. But if their grade is below 60, we move to the inner “else” condition, which gives the student the option to retake the exam. If they say yes, we wish them good luck on the retake. But if they say no, we tell them to try again next semester.
Â
See how we can build upon the basic structure of “if-else” statements to create more complex decision-making logic? However, it’s important to keep in mind that as we add more layers of nested statements, the code can become harder to read and maintain. So, use nested “if-else” statements wisely!
Â
Â
Let’s talk about “if-elif-else” statements, also known as a “switch case” in other programming languages.
Â
Imagine you’re playing a game where you have to choose a character to play with. Each character has their own set of abilities and strengths, and you want to make sure the player selects a valid character. That’s where “if-elif-else” statements can help!
Â
Think of it like a flowchart, where each decision leads you down a different path. You start with the initial “if” condition, and if that’s not true, you move on to the first “elif” condition. If that’s not true either, you move on to the next “elif” condition, and so on, until you reach the final “else” condition.
Â
Let’s take a look at an example:
In this example, we start with the initial “if” condition to check if the player selected the Warrior character. If that’s not true, we move on to the first “elif” condition to check if the player selected the Mage character. If that’s not true either, we move on to the next “elif” condition to check if the player selected the Rogue character. Finally, if none of these conditions are true, we reach the “else” condition and print an error message.
“if-elif-else” statements can be incredibly useful when you need to check multiple conditions and choose a different outcome for each one. However, be careful not to create too many “elif” conditions, as this can make your code hard to read and understand.
Have you ever found yourself writing long if-else statements just to assign a value to a variable? It can be tedious and sometimes difficult to read. That’s where the Ternary Operator comes in!
Â
The Ternary Operator is a shorthand way of writing an if-else statement in one line of code. It’s also sometimes referred to as a “conditional expression”. Here’s an example:
Â
Code using a long if-else statement:
Now let us remake the same code using ternary operator
As you can see, the Ternary Operator allows us to assign a value to the variable “y” based on a condition in a single line of code. The syntax is:
Â
value_if_true if condition else value_if_false
In the example above, if the condition “x > 5” is True, then the value “greater than 5” is assigned to the variable “y”. If the condition is False, then the value “less than or equal to 5” is assigned.
Â
The Ternary Operator can be a great way to simplify your code and make it more readable. However, be careful not to overuse it. In some cases, a long if-else statement may be more appropriate for the situation.
In this section, we’ll take a look at some common programs that utilize conditional statements. These exercises will provide you with a solid foundation and help you build your logic skills. While we will provide solutions at the end of the blog, we encourage you to try to solve these exercises on your own first. Don’t worry if you don’t get it right the first time, practice makes perfect
Â
Exercise 1:Â
Write a program that takes a number from the user (between 0 to 9) and prints that number in word format. For example, if the user inputs “5”, the program should output “five”. Can you implement this using conditional statements in Python?
Â
Exercise 2:Â
Write a program that takes three numbers from the user and finds the maximum number out of those three. You can use conditional statements to compare the numbers and determine which one is the largest. Remember to take input from the user using input() and convert it to integers using int() before performing any comparisons.
Â
Exercise 3:Â
Design a calculator that takes two numbers as input from the user and prompts the user to enter an operation code (e.g., +, -, *, /).Â
Based on the operation code entered, perform the appropriate mathematical operation on the two numbers and display the result to the user
Â
Exercise 4:Â
Write a Python program to check whether a given year is a leap year or not. Ask the user to enter the year and then check if it is a leap year or not. If the year is a leap year, print “Leap year”. Otherwise, print “Not a leap year”.
Note: A leap year is a year that is divisible by 4 but not divisible by 100, unless it is also divisible by 400.
Â
Exercise 5:Â
Write a Python program to check if a given string is a Palindrome or not.
Hint: A string is said to be Palindrome if the reverse of the string is same as string itself.
Example: Input: “racecar” Output: “Given string is Palindrome.”
Input: “hello” Output: “Given string is not Palindrome.”
And that’s a wrap on Conditional Statements in Python! We’ve covered the basics of “if” statements, “if-else” statements, “if-elif-else” statements, and even the Ternary Operator. With these tools in your toolkit, you’ll be able to make decisions in your code and create more dynamic programs.
Â
But don’t stop here! Make sure to check out our previous blogs in our Python series where we’ve discussed operators and data types. And in our next installment, we’ll be diving into loop statements in Python. Stay tuned!
Â
Remember, programming is a journey, and we’re excited to be on this journey with you. Keep practicing, keep experimenting, and keep having fun and follow 1stepgrow.
Solution 1:
Note that we use input() to take the user’s input as a string, and then convert it to an integer using int() so we can perform numeric comparisons. We then use a series of if-elif statements to check which number was inputted and print it in word format. If the input is not a number between 0 to 9, we print an error message.
Â
Solution 2:
In this solution, we first take input from the user using input() and convert it to integers using int(). Then we use conditional statements (if, elif, and else) to compare the numbers and determine which one is the largest. Finally, we print out the maximum number using print().
Â
Solution 3:
Note that in this solution, we take two numbers and the operator from the user using the input() function. Then we use conditional statements to check which operator was entered and perform the appropriate mathematical operation. Finally, we display the result to the user using formatted string literals (f-strings).
Â
Solution 4:
In this program, we first take the input year from the user using the input() function. Then, we use the modulo operator % to check if the year is divisible by 4 and not divisible by 100, or if it is divisible by 400. If either of these conditions is true, we print a message saying that the year is a leap year. Otherwise, we print a message saying that the year is not a leap year.
Â
Solution 5:
In this solution, we take the input from the user and remove any spaces in the input string. Then, we use a conditional statement to check if the string is a palindrome by comparing the original string to its reverse. If they are the same, we print a message saying that the string is a palindrome, otherwise we print a message saying that it is not a palindrome.
We provide online certification in Data Science and AI, Digital Marketing, Data Analytics with a job guarantee program. For more information, contact us today!
Courses
1stepGrow
Anaconda | Jupyter Notebook | Git & GitHub (Version Control Systems) | Python Programming Language | R Programming Langauage | Linear Algebra & Statistics | ANOVA | Hypothesis Testing | Machine Learning | Data Cleaning | Data Wrangling | Feature Engineering | Exploratory Data Analytics (EDA) | Â ML Algorithms | Linear Regression | Logistic Regression | Decision Tree | Random Forest | Bagging & Boosting | PCA | SVM | Â Time Series Analysis | Natural Language Processing (NLP) | NLTK | Deep Learning | Neural Networks | Computer Vision | Reinforcement Learning | ANN | CNN | RNN | LSTM | Facebook Prophet | SQL | MongoDB | Advance Excel for Data Science | BI Tools | Tableau | Power BI | Big Data | Hadoop | Apache Spark | Azure Datalake | Cloud Deployment | AWS | GCP | AGILE & SCRUM | Data Science Capstone Projects | ML Capstone Projects | AI Capstone Projects | Domain Training | Business Analytics
WordPress | Elementor | On-Page SEO | Off-Page SEO | Technical SEO | Content SEO | SEM | PPC | Social Media Marketing | Email Marketing | Inbound Marketing | Web Analytics | Facebook Marketing | Mobile App Marketing | Content Marketing | YouTube Marketing | Google My Business (GMB) | CRM | Affiliate Marketing | Influencer Marketing | WordPress Website Development | AI in Digital Marketing | Portfolio Creation for Digital Marketing profile | Digital Marketing Capstone Projects
Jupyter Notebook | Git & GitHub | Python | Linear Algebra & Statistics | ANOVA | Hypothesis Testing | Machine Learning | Data Cleaning | Data Wrangling | Feature Engineering | Exploratory Data Analytics (EDA) | Â ML Algorithms | Linear Regression | Logistic Regression | Decision Tree | Random Forest | Bagging & Boosting | PCA | SVM | Â Time Series Analysis | Natural Language Processing (NLP) | NLTK | SQL | MongoDB | Advance Excel for Data Science | Alteryx | BI Tools | Tableau | Power BI | Big Data | Hadoop | Apache Spark | Azure Datalake | Cloud Deployment | AWS | GCP | AGILE & SCRUM | Data Analytics Capstone Projects
Anjanapura | Arekere | Basavanagudi | Basaveshwara Nagar | Begur | Bellandur | Bommanahalli | Bommasandra | BTM Layout | CV Raman Nagar | Electronic City | Girinagar | Gottigere | Hebbal | Hoodi | HSR Layout | Hulimavu | Indira Nagar | Jalahalli | Jayanagar | J. P. Nagar |Â Kamakshipalya | Kalyan Nagar | Kammanahalli | Kengeri | Koramangala | Kothnur | Krishnarajapuram | Kumaraswamy Layout | Lingarajapuram | Mahadevapura | Mahalakshmi Layout | Malleshwaram | Marathahalli | Mathikere | Nagarbhavi | Nandini Layout | Nayandahalli | Padmanabhanagar | Peenya | Pete Area | Rajaji Nagar | Rajarajeshwari Nagar | Ramamurthy Nagar | R. T. Nagar | Sadashivanagar | Seshadripuram | Shivajinagar | Ulsoor | Uttarahalli | Varthur | Vasanth Nagar | Vidyaranyapura | Vijayanagar | White Field | Yelahanka | Yeshwanthpur
Mumbai | Pune | Nagpur | Delhi | Gurugram | Chennai | Hyderabad | Coimbatore | Bhubaneswar | Kolkata | Indore | Jaipur and More