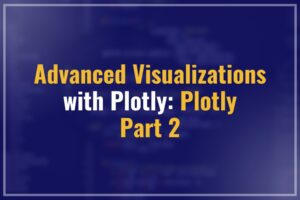
11-13 Months | 28 Projects
7-8 Months | 14 Projects
6-7 Months | 12 Projects
4.5 Months | 45 Projects
11-13 Months | 28 Projects
7-8 Months | 14 Projects
9-11 Months | 24 Projects
8-10 Months | 20 Projects
8-10 Months | 20 Projects
6-7 Months | 12 Projects
3-4 Months | 6 Projects
4.5 Months | 45+ Projects
3 Months | 30 Projects
11-13 Months | 28 Projects
7-8 Months | 14 Projects
9-11 Months | 24 Projects
8-10 Months | 20 Projects
8-10 Months | 20 Projects
6-7 Months | 12 Projects
3-4 Months | 6 Projects
4.5 Months | 45+ Projects
3 Months | 30 Projects
Welcome to the eagerly awaited second part of our journey into the captivating world of regular expressions. In this part of Advanced Regular expressions, we’re diving even deeper into the intricacies and versatility of text pattern matching. Building upon the foundational knowledge from Part 1, we’ll unravel more advanced topics that will empower you to tackle complex text manipulation tasks with confidence.
Get ready to explore a range of exciting concepts that will elevate your understanding of regular expressions to new heights:
First let us take a look at some of the functions used in regular expressions
Embark on a journey through the world of advanced regular expression functions. Within the domain of regular expressions, the Python re module offers a collection of potent functions. These functions empower you to seamlessly search, extract, and manipulate data by leveraging distinct patterns. Delve into the diverse functionalities that these functions bring to the table, allowing you to wield text manipulation with utmost precision and ease.
The search() function within the regular expression module empowers you to uncover the first occurrence of a regular expression pattern in a given string. This function returns a match object, providing access to the matched content through the group() method. Additionally, you can retrieve the starting and ending indices of the match using the span() method.
re.search(regex, look_up_string)
Consecutively we can use .span() and .group() which will return the index value and the actual value of the matched strings. Let us understand this with the help of an example.
The match() function, a feature of the re module, serves as a tool to identify whether a given regular expression pattern exists at the beginning of a string. When successful, this function returns a match object, which can be accessed using the group() method to extract the matched content. Conversely, if the pattern is not found at the beginning, the function returns None.
re.match(regex, look_up_string)
Consecutively we can use .span() and .group() which will return the index value and the actual value of the matched strings. Let us understand this with the help of an example.
In this example, the match() function detects a sequence of digits at the beginning of the text. The group() method extracts the matched content (“42”), while the span() method gives the index span of the match (from index 0 to 1). If the pattern doesn’t appear at the start of the string, the function returns None, indicating no match.
The findall() function within the re module is a remarkable tool for unveiling the hidden treasures that lie within your text. With its ability to return all non-overlapping matches of a pattern, it transforms the process of extracting data from strings into a breeze.
re.findall(regex, look_up_string)
Let’s unravel the power of findall() with a practical code example:
In this example, we invoke the split() function to gracefully disassemble the text into fragments, using the , followed by optional whitespace as the splitting pattern. The result is an eloquent list of individual fruit names, emphasizing the function’s aptitude for breaking down strings into meaningful components.
The sub() function, a remarkable feature within the re module, invites you to perform remarkable transformations upon strings. By identifying and replacing the leftmost non-overlapping occurrences of a specified pattern in a given string, sub() empowers you to substitute and reshape text with unparalleled ease.
re.sub(regex, ‘new_string’, look_up_string)
In this example, we invoke the sub() function to replace the occurrence of ‘dear’ with ‘beloved’ in the text. The result is a transformed string, a testament to the function’s ability to reshape text in a way that suits your requirements.
In this segment of our journey through regular expressions, we’re about to delve into the world of alternation and groups. Alternation, denoted by the vertical bar |, empowers you to explore multiple possibilities within a pattern, enabling you to match any one of the provided options. On the other hand, groups allow you to establish order within your patterns, simplifying complex expressions and enhancing the control you have over matching and capturing text.
When dealing with text patterns that offer multiple possibilities, the R|S construct, known as alternation, emerges as a potent solution. Alternation empowers you to indicate that either pattern R or pattern S can be considered a successful match. This versatility proves invaluable when seeking variations within your text data.
Using the R|S pattern, you can create a choice between alternative patterns. This implies that if either R or S is found in the text, the match is achieved.
re.search(r’R|S’, look_up_string)
Let’s explore alternation through an illustrative code example:
In this example, the search() function uses alternation to match either ‘color’ or ‘colour’ in the text. The first occurrence of either variant is returned as the result. Alternation is a powerful technique for handling variations in text data efficiently and comprehensively.
Consider a scenario where you want to identify if a sentence contains either the word “apple” or “banana.” We can achieve this using the alternation construct R|S.
In this example, we utilize the alternation pattern apple|banana to search for occurrences of either “apple” or “banana” within the sentence. The result highlights the beauty of alternation, allowing us to cater to various word choices effortlessly. Whether it’s apples, bananas, or other text variations, alternation empowers us to identify and work with different options efficiently.
In the realm of regular expressions, capturing groups offer a sophisticated way to not only identify specific segments within text but also capture these segments for later use. By enclosing portions of your pattern in parentheses ( ), you not only delineate distinct sections but also enable the extraction of these sections through backreferences.
re.search(r'(pattern)’, look_up_string)
re.search(r’\1′, look_up_string)
In this example, we use a capturing group (.*?) to capture the weather condition. The parentheses indicate the group, while the .*? captures any characters within it. The backreference group(1) retrieves the content of the captured group. Capturing groups and backreferences are powerful tools that allow you to extract specific segments of interest within your text for further analysis or manipulation.
In this example, we capture both the fruit name and its corresponding price using capturing groups. The backreferences group(1) and group(2) enable us to retrieve and display these captured segments. This showcases how capturing groups and backreferences empower you to extract and manipulate specific information from your text data with elegance and precision.
In the realm of regular expressions, precision and control over patterns are paramount. Non-capturing groups, represented by (?: ), offer an elegant solution when you need to create groups for purposes such as alternation or applying quantifiers, without necessarily capturing the matched content. These groups enhance your ability to craft intricate patterns while maintaining flexibility and readability.
Non-Capturing Groups: Denoted by (?: ), these groups allow you to group patterns without capturing the matched content. This is particularly useful when you want to apply quantifiers or alternation, but you don’t need to store the matched content for later use.
re.search(r'(?:pattern)’, look_up_string)
In this example, we utilize a non-capturing group (?:colou?r) to match both “color” and “colour” variations. The (?: ) ensures that the matched content isn’t captured, allowing us to focus on matching the variations while avoiding unnecessary group captures. This highlights the elegance of non-capturing groups in crafting patterns that balance complexity and clarity.
Welcome to the section where we dive into the realm of advanced regular expressions. Having established a strong foundation with the basics, it’s time to elevate your understanding and mastery of regex to new heights.
In the world of advanced regular expressions, precision is often the key to unravelling complex text patterns. The {m,n} quantifier provides you with the ability to specify a specific range of occurrences for a pattern. This granular control allows you to precisely match text that adheres to your desired repetition criteria.
re.search(r’pattern{m,n}’, look_up_string)
Let’s explore the {m,n} quantifier with an illustrative example:
In this example, the regular expression \b\d{2,4}\b matches numbers with 2 to 4 digits. The output displays both numbers, “1234” and “12,” that satisfy this range condition. The \b word boundaries ensure that complete numbers are captured.
In the realm of advanced regular expressions, efficiency and precision often walk hand in hand. The non-greedy versions of quantifiers provide you with the ability to capture text in a minimalistic manner. By default, quantifiers are greedy, aiming to match as much text as possible. Non-greedy quantifiers, on the other hand, aim for the shortest possible match, ensuring that your patterns capture the least amount of text necessary.
re.search(r’pattern*?’, look_up_string) # non-Greedy ‘*’ quantifier
Here, (.*?) non-greedy quantifiers capture individual descriptions of the animals. The result showcases the finesse of non-greedy quantifiers, ensuring that each description is succinctly captured. A greedy quantifier would seize the entire text between the first “a” and the last “.”, resulting in a single match.
With non-greedy quantifiers, you attain surgical precision in extracting essential fragments, elevating your text manipulation prowess to new heights.
In the labyrinth of regular expressions, the power of foresight and hindsight lies within lookaheads and lookbehinds. These assertions provide the capability to match patterns based on conditions that occur before or after the text you’re targeting. Lookaheads enable you to explore what lies ahead, while lookbehinds delve into what came before. This advanced technique elevates your text processing to a new level of sophistication.
In this example, the positive lookahead (?=\,) is used to match email addresses that are followed by a comma. The negative lookbehind (?<!Email: ) ensures that phone numbers are captured only if they are not preceded by ‘Email:’. These assertions enable you to extract precisely the information you need by considering the context in which it appears.
By harnessing the potential of lookaheads and lookbehinds, you transcend the boundaries of simple pattern matching, delving into the realm of contextual awareness for advanced text manipulation.
In this practical segment, we venture into the real-world applications of advanced regular expressions. Armed with the knowledge you’ve gained so far, we’ll explore how regular expressions can solve common challenges encountered in text processing.
Regular expressions are invaluable tools for validating user input, such as email addresses and URLs. By defining specific patterns, you can ensure that the data entered conforms to the expected format.
This example showcases how regular expressions help validate email addresses by enforcing a specific format that includes a valid username, domain name, and TLD. It demonstrates the power of regex in ensuring that user-provided data adheres to defined patterns, enhancing data integrity and accuracy.
As we conclude our exploration of advanced regular expressions, you’ve gained a potent toolset for text manipulation. We navigated from syntax basics to advanced techniques like lookaheads, lookbehinds, and non-greedy quantifiers. These concepts empower precise text extraction, validation, and formatting.
From validating emails and URLs to extracting data and refining text, you’ve witnessed the versatility of regular expressions in action. Remember, practice is your companion on the journey to mastering this skill. Regular expressions enhance your data tasks, boost text analysis, and automate processes. follow 1stepgrow for more blogs.
We provide online certification in data science and AI, digital marketing, data analytics with a job guarantee program. For more information, contact us today!
Courses
1stepGrow
Anaconda | Jupyter Notebook | Git & GitHub (Version Control Systems) | Python Programming Language | R Programming Langauage | Linear Algebra & Statistics | ANOVA | Hypothesis Testing | Machine Learning | Data Cleaning | Data Wrangling | Feature Engineering | Exploratory Data Analytics (EDA) | ML Algorithms | Linear Regression | Logistic Regression | Decision Tree | Random Forest | Bagging & Boosting | PCA | SVM | Time Series Analysis | Natural Language Processing (NLP) | NLTK | Deep Learning | Neural Networks | Computer Vision | Reinforcement Learning | ANN | CNN | RNN | LSTM | Facebook Prophet | SQL | MongoDB | Advance Excel for Data Science | BI Tools | Tableau | Power BI | Big Data | Hadoop | Apache Spark | Azure Datalake | Cloud Deployment | AWS | GCP | AGILE & SCRUM | Data Science Capstone Projects | ML Capstone Projects | AI Capstone Projects | Domain Training | Business Analytics
WordPress | Elementor | On-Page SEO | Off-Page SEO | Technical SEO | Content SEO | SEM | PPC | Social Media Marketing | Email Marketing | Inbound Marketing | Web Analytics | Facebook Marketing | Mobile App Marketing | Content Marketing | YouTube Marketing | Google My Business (GMB) | CRM | Affiliate Marketing | Influencer Marketing | WordPress Website Development | AI in Digital Marketing | Portfolio Creation for Digital Marketing profile | Digital Marketing Capstone Projects
Jupyter Notebook | Git & GitHub | Python | Linear Algebra & Statistics | ANOVA | Hypothesis Testing | Machine Learning | Data Cleaning | Data Wrangling | Feature Engineering | Exploratory Data Analytics (EDA) | ML Algorithms | Linear Regression | Logistic Regression | Decision Tree | Random Forest | Bagging & Boosting | PCA | SVM | Time Series Analysis | Natural Language Processing (NLP) | NLTK | SQL | MongoDB | Advance Excel for Data Science | Alteryx | BI Tools | Tableau | Power BI | Big Data | Hadoop | Apache Spark | Azure Datalake | Cloud Deployment | AWS | GCP | AGILE & SCRUM | Data Analytics Capstone Projects
Anjanapura | Arekere | Basavanagudi | Basaveshwara Nagar | Begur | Bellandur | Bommanahalli | Bommasandra | BTM Layout | CV Raman Nagar | Electronic City | Girinagar | Gottigere | Hebbal | Hoodi | HSR Layout | Hulimavu | Indira Nagar | Jalahalli | Jayanagar | J. P. Nagar | Kamakshipalya | Kalyan Nagar | Kammanahalli | Kengeri | Koramangala | Kothnur | Krishnarajapuram | Kumaraswamy Layout | Lingarajapuram | Mahadevapura | Mahalakshmi Layout | Malleshwaram | Marathahalli | Mathikere | Nagarbhavi | Nandini Layout | Nayandahalli | Padmanabhanagar | Peenya | Pete Area | Rajaji Nagar | Rajarajeshwari Nagar | Ramamurthy Nagar | R. T. Nagar | Sadashivanagar | Seshadripuram | Shivajinagar | Ulsoor | Uttarahalli | Varthur | Vasanth Nagar | Vidyaranyapura | Vijayanagar | White Field | Yelahanka | Yeshwanthpur
Mumbai | Pune | Nagpur | Delhi | Gurugram | Chennai | Hyderabad | Coimbatore | Bhubaneswar | Kolkata | Indore | Jaipur and More