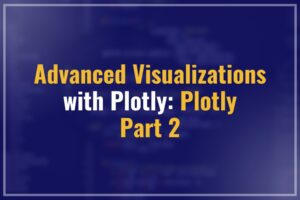
11-13 Months | 28 Projects
7-8 Months | 14 Projects
6-7 Months | 12 Projects
4.5 Months | 45 Projects
11-13 Months | 28 Projects
7-8 Months | 14 Projects
9-11 Months | 24 Projects
8-10 Months | 20 Projects
8-10 Months | 20 Projects
6-7 Months | 12 Projects
3-4 Months | 6 Projects
4.5 Months | 45+ Projects
3 Months | 30 Projects
11-13 Months | 28 Projects
7-8 Months | 14 Projects
9-11 Months | 24 Projects
8-10 Months | 20 Projects
8-10 Months | 20 Projects
6-7 Months | 12 Projects
3-4 Months | 6 Projects
4.5 Months | 45+ Projects
3 Months | 30 Projects
Welcome to part 4 of our Python series! In this post, we’ll be diving into the world of numeric data types in Python. From integers to floats and complex numbers, we’ll cover everything you need to know about Python’s numeric data types in one comprehensive guide. We’ll also explore numeric data type conversions and show you how to convert between different numeric types. So, sit tight, grab a cup of coffee, and get ready to master Python’s numeric data types!
Numeric data types in Python are data types that represent numerical values, such as integers, floating-point numbers, and complex numbers. These data types are used extensively in programming for performing mathematical calculations and numerical computations. Understanding the characteristics and usage of the different numeric data types in Python is essential for writing accurate and efficient programs.
As we have discussed earlier, there are three types of numeric data types in Python: integers, floats, and complex numbers. These data types have the following characteristics:
Let’s take a closer look at each of these data types one by one and explore their features and usage in Python.
Integers are the building blocks of mathematics; they are the simplest type of numbers with no fractional component. And in Python, integers are the most basic numeric data type that you can work with. They are represented by the ‘int’ class and are capable of storing both positive and negative values, including zero.
But what’s interesting is that Python has its own limits on the range of integers that can be represented. On a 32-bit system, the minimum and maximum values for integers are -2^31 and 2^31-1 respectively, while on a 64-bit system, the range is much wider with the minimum and maximum values being -2^63 and 2^63-1 respectively. With these characteristics, integers provide a solid foundation for performing all sorts of mathematical operations in Python.
One characteristic of integers in Python is that they are immutable, meaning that once an integer is created, its value cannot be changed. Integers in Python support various arithmetic and bitwise operations, as well as comparison and logical operators.
Here is an example of how we can perform arithmetic operations on integers:
Exercise: Write a Python program that takes two integers as input from the user and calculates their sum, difference, product, and quotient. Print the results to the console.
Hint: You can use the input() function to take user input. However, even if the input is an integer, the input() function will treat it as a string. To convert the input to an integer, you can use the int() function like this: x = int(input()).
A floating-point number, or float, is a type of numeric data type in Python that represents a real number with a fractional component. In other words, floats are used to represent numbers that are not integers, such as 3.14 or -2.5.
Floats in Python are represented by the ‘float’ class and are stored in a fixed amount of memory. They have a significant precision and can represent a wide range of values, including very large or very small numbers.
One thing to keep in mind when working with floats is that they are not always exact due to the way they are stored in memory. This can sometimes lead to unexpected results when performing mathematical operations on floats, especially when working with very small or very large values. Therefore, it’s important to be careful when working with floats and to be aware of potential precision errors.
For example, when we try to add 0.1 and 0.2 in Python, we might expect the result to be 0.3. However, due to precision errors, the result of this operation may actually be something like 0.30000000000000004. This might not seem like a big deal, but it can lead to significant errors in calculations that rely on precise values.
Here is an example of how you can use floating point data type in your python program:
Floating-point numbers in Python support all the same arithmetic operations, logical operations, bitwise operations, and comparison operations as integers. However, as mentioned earlier, precision errors can occur when working with floating-point numbers, which can sometimes lead to unexpected results. Therefore, it’s important to be careful when working with floats and to be aware of potential precision errors.
Handling precision and rounding errors in floating point data type:
3. Be aware of limitations: It’s important to be aware of the limitations of floating-point numbers and the potential for precision and rounding errors. When working with very small or very large values, it may be necessary to use alternative data types or libraries to ensure accurate results.
Exercise: Calculating the Area of a Circle
Write a program that takes the radius of a circle as input from the user and calculates the area of the circle. Display the result rounded to two decimal places.
A complex data type is used to represent complex numbers, which have a real part and an imaginary part. In Python, complex numbers are represented using the complex class, with the imaginary part denoted using the letter “j“. For example, 3 + 4j is a complex number with a real part of 3 and an imaginary part of 4.
Similar to integers and floats, complex numbers are also immutable, which means that once a complex number is created, its value cannot be changed.
Here is an example demonstrating the use of complex data type in python:
These are the three basic numeric data types in python, now let us look at numeric data type conversion in python.
Numeric data type conversions in Python refer to the process of converting one type of numeric data to another type. In Python, there are several types of numeric data types such as integers, floating-point numbers, and complex numbers as we have discussed earlier. Sometimes it may be necessary to convert one data type to another to perform arithmetic operations or to compare values. Python provides various functions and methods to convert numeric data types. In this section, we will discuss the various ways to perform numeric data type conversions in Python.
In Python, there are two types of numeric data type conversions:
In Python, it’s often necessary to convert one numeric data type to another. This can be done using built-in functions or by explicitly casting the data type.
The most common numeric data type conversions in Python are:
2. Float to Integer: This conversion is done using the int() function. Note that the decimal part of the float will be truncated. For example:
3. Float to Complex: This conversion is done using the complex() function. The real part of the complex number will be the float value and the imaginary part will be 0. For example:
4. Integer to Complex: This conversion is done using the complex() function. The real part of the complex number will be the integer value and the imaginary part will be 0. For example:
Implicit conversions occur when values of different data types are used together in an operation. For example, when we add an integer and a float, the integer is implicitly converted to a float before the addition is performed.
It’s important to be aware of the potential loss of precision that can occur during type conversions, especially when converting between float and integer data types.
Let’s take a look at some examples of implicit conversions in Python:
Congratulations on making it to the end of our blog on numeric data types in Python! We hope that you found this article informative and that you gained a good understanding of the different numeric data types in Python, as well as how to convert between them. Stay tuned for our next post and follow 1StepGrow where we will be diving into the world of string data type in Python. Thanks for taking the time to read our blog and happy coding!
We provide online certification in data science and AI, digital marketing, data analytics with a job guarantee program. For more information, contact us today!
Courses
1stepGrow
Anaconda | Jupyter Notebook | Git & GitHub (Version Control Systems) | Python Programming Language | R Programming Langauage | Linear Algebra & Statistics | ANOVA | Hypothesis Testing | Machine Learning | Data Cleaning | Data Wrangling | Feature Engineering | Exploratory Data Analytics (EDA) | ML Algorithms | Linear Regression | Logistic Regression | Decision Tree | Random Forest | Bagging & Boosting | PCA | SVM | Time Series Analysis | Natural Language Processing (NLP) | NLTK | Deep Learning | Neural Networks | Computer Vision | Reinforcement Learning | ANN | CNN | RNN | LSTM | Facebook Prophet | SQL | MongoDB | Advance Excel for Data Science | BI Tools | Tableau | Power BI | Big Data | Hadoop | Apache Spark | Azure Datalake | Cloud Deployment | AWS | GCP | AGILE & SCRUM | Data Science Capstone Projects | ML Capstone Projects | AI Capstone Projects | Domain Training | Business Analytics
WordPress | Elementor | On-Page SEO | Off-Page SEO | Technical SEO | Content SEO | SEM | PPC | Social Media Marketing | Email Marketing | Inbound Marketing | Web Analytics | Facebook Marketing | Mobile App Marketing | Content Marketing | YouTube Marketing | Google My Business (GMB) | CRM | Affiliate Marketing | Influencer Marketing | WordPress Website Development | AI in Digital Marketing | Portfolio Creation for Digital Marketing profile | Digital Marketing Capstone Projects
Jupyter Notebook | Git & GitHub | Python | Linear Algebra & Statistics | ANOVA | Hypothesis Testing | Machine Learning | Data Cleaning | Data Wrangling | Feature Engineering | Exploratory Data Analytics (EDA) | ML Algorithms | Linear Regression | Logistic Regression | Decision Tree | Random Forest | Bagging & Boosting | PCA | SVM | Time Series Analysis | Natural Language Processing (NLP) | NLTK | SQL | MongoDB | Advance Excel for Data Science | Alteryx | BI Tools | Tableau | Power BI | Big Data | Hadoop | Apache Spark | Azure Datalake | Cloud Deployment | AWS | GCP | AGILE & SCRUM | Data Analytics Capstone Projects
Anjanapura | Arekere | Basavanagudi | Basaveshwara Nagar | Begur | Bellandur | Bommanahalli | Bommasandra | BTM Layout | CV Raman Nagar | Electronic City | Girinagar | Gottigere | Hebbal | Hoodi | HSR Layout | Hulimavu | Indira Nagar | Jalahalli | Jayanagar | J. P. Nagar | Kamakshipalya | Kalyan Nagar | Kammanahalli | Kengeri | Koramangala | Kothnur | Krishnarajapuram | Kumaraswamy Layout | Lingarajapuram | Mahadevapura | Mahalakshmi Layout | Malleshwaram | Marathahalli | Mathikere | Nagarbhavi | Nandini Layout | Nayandahalli | Padmanabhanagar | Peenya | Pete Area | Rajaji Nagar | Rajarajeshwari Nagar | Ramamurthy Nagar | R. T. Nagar | Sadashivanagar | Seshadripuram | Shivajinagar | Ulsoor | Uttarahalli | Varthur | Vasanth Nagar | Vidyaranyapura | Vijayanagar | White Field | Yelahanka | Yeshwanthpur
Mumbai | Pune | Nagpur | Delhi | Gurugram | Chennai | Hyderabad | Coimbatore | Bhubaneswar | Kolkata | Indore | Jaipur and More